2019-09-25 03:45:59 +10:00
< h1 align = "center" > < img width = "440" src = "docs/crossterm_full.png" / > < / h1 >
2019-09-26 00:09:16 +10:00
[](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick& hosted_button_id=Z8QK6XU749JB2) ![Travis][s7] [![Latest Version][s1]][l1] [![MIT][s2]][l2] [![docs][s3]][l3] ![Lines of Code][s6] [![Join us on Discord][s5]][l5]
2018-07-15 07:37:21 +10:00
2019-10-02 16:49:01 +10:00
# Cross-platform Terminal Manipulation Library
2019-09-13 05:39:39 +10:00
2019-10-02 16:49:01 +10:00
Have you ever been disappointed when a terminal library for the Rust language was only written for UNIX systems?
Crossterm provides clearing, input handling, styling, cursor movement and terminal actions for both
2019-09-26 00:09:16 +10:00
Windows and UNIX systems.
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
Crossterm aims to be simple and easy to call in code. Through the simplicity of Crossterm, you do not have to
worry about the platform you are working with.
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
This crate supports all UNIX and Windows terminals down to Windows 7 (not all terminals are tested,
2019-09-26 00:09:16 +10:00
see [Tested Terminals ](#tested-terminals ) for more info).
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
## Table of Contents
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
* [Features ](#features )
* [Tested Terminals ](#tested-terminals )
* [Getting Started ](#getting-started )
* [Feature Flags ](#feature-flags )
* [`crossterm` vs `crossterm_*` crates ](#crossterm-vs-crossterm_-crates )
* [Other Resources ](#other-resources )
* [Used By ](#used-by )
* [Contributing ](#contributing )
2018-07-31 05:35:35 +10:00
2018-09-23 06:55:30 +10:00
## Features
2018-07-31 05:35:35 +10:00
2018-11-26 00:17:11 +11:00
- Cross-platform
2019-09-26 00:09:16 +10:00
- Multi-threaded (send, sync)
2019-10-02 16:49:01 +10:00
- Detailed documentation
- Few dependencies
- Cursor (feature `cursor` )
- Move the cursor N times (up, down, left, right)
- Set/get the cursor position
- Store the cursor position and restore to it later
- Hide/show the cursor
- Enable/disable cursor blinking (not all terminals do support this feature)
- Styled output (feature `style` )
- Foreground color (16 base colors)
- Background color (16 base colors)
- 256 (ANSI) color support (Windows 10 and UNIX only)
- RGB color support (Windows 10 and UNIX only)
- Text attributes like bold, italic, underscore, crossed, etc.
- Terminal (feature `terminal` )
- Clear (all lines, current line, from cursor down and up, until new line)
- Scroll up, down
- Set/get the terminal size
- Exit current process
- Input (feature `input` )
2018-08-15 05:40:07 +10:00
- Read character
- Read line
2019-04-11 07:46:30 +10:00
- Read key input events (async / sync)
- Read mouse input events (press, release, position, button)
2019-10-02 16:49:01 +10:00
- Screen (feature `screen` )
- Alternate screen
- Raw screen
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
<!--
WARNING: Do not change following heading title as it's used in the URL by other crates!
-->
2019-03-22 02:00:30 +11:00
2019-10-02 16:49:01 +10:00
### Tested Terminals
2019-09-26 00:09:16 +10:00
2019-10-02 16:49:01 +10:00
- Windows Powershell
- Windows 10 (Pro)
- Windows CMD
- Windows 10 (Pro)
- Windows 8.1 (N)
- Ubuntu Desktop Terminal
- Ubuntu 17.10
- (Arch, Manjaro) KDE Konsole
- Linux Mint
2019-03-22 02:00:30 +11:00
2019-10-02 16:49:01 +10:00
This crate supports all UNIX terminals and Windows terminals down to Windows 7; however, not all of the
terminals have been tested. If you have used this library for a terminal other than the above list without
issues, then feel free to add it to the above list - I really would appreciate it!
2019-03-22 02:00:30 +11:00
2019-10-02 16:49:01 +10:00
## Getting Started
2019-09-26 00:09:16 +10:00
2019-10-02 16:49:01 +10:00
< details >
< summary >
Click to show Cargo.toml.
< / summary >
2019-03-22 02:00:30 +11:00
2019-10-02 16:49:01 +10:00
```toml
[dependencies]
crossterm = "0.11"
2019-03-22 02:00:30 +11:00
```
2019-09-26 00:09:16 +10:00
2019-10-02 16:49:01 +10:00
< / details >
< p > < / p >
2019-09-26 00:09:16 +10:00
2019-03-22 02:00:30 +11:00
```rust
2019-10-02 16:49:01 +10:00
use std::io::{stdout, Write};
use crossterm::{execute, Attribute, Color, Output, ResetColor, Result, SetBg, SetFg};
fn main() -> Result< ()> {
execute!(
stdout(),
// Blue foreground
SetFg(Color::Blue),
// Red background
SetBg(Color::Red),
Output("Styled text here.".to_string()),
// Reset to default colors
ResetColor
)
}
2018-07-31 05:35:35 +10:00
```
2019-03-22 02:00:30 +11:00
2019-10-02 16:49:01 +10:00
### Feature Flags
2019-09-26 00:09:16 +10:00
2019-10-02 16:49:01 +10:00
All features are enabled by default. You can disable default features and enable some of them only.
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
```toml
[dependencies.crossterm]
version = "0.11"
default-features = false # Disable default features
features = ["cursor", "screen"] # Enable required features only
2018-07-31 05:35:35 +10:00
```
2019-10-02 16:49:01 +10:00
| Feature | Description | Links |
| :-- | :-- | :-- |
| `input` | Sync/Async input readers | [API documentation ](https://docs.rs/crossterm_input ), [crates.io ](https://crates.io/crates/crossterm_input ), [GitHub ](https://github.com/crossterm-rs/crossterm-input ) |
| `cursor` | Cursor manipulation | [API documentation ](https://docs.rs/crossterm_cursor ), [crates.io ](https://crates.io/crates/crossterm_cursor ), [GitHub ](https://github.com/crossterm-rs/crossterm-cursor ) |
| `screen` | Alternate screen & raw mode | [API documentation ](https://docs.rs/crossterm_screen ), [crates.io ](https://crates.io/crates/crossterm_screen ), [GitHub ](https://github.com/crossterm-rs/crossterm-screen ) |
| `terminal` | Size, clear, scroll | [API documentation ](https://docs.rs/crossterm_terminal ), [crates.io ](https://crates.io/crates/crossterm_terminal ), [GitHub ](https://github.com/crossterm-rs/crossterm-terminal ) |
| `style` | Colors, text attributes | [API documentation ](https://docs.rs/crossterm_style ), [crates.io ](https://crates.io/crates/crossterm_style ), [GitHub ](https://github.com/crossterm-rs/crossterm-style ) |
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
### `crossterm` vs `crossterm_*` crates
2019-03-11 10:09:41 +11:00
2019-10-02 16:49:01 +10:00
There're two ways how to use the Crossterm library:
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
* `crossterm` crate with the `cursor` feature flag,
* `crossterm_cursor` crate.
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
Both provide same functionality, the only difference is the namespace (`crossterm` vs `crossterm_cursor` ).
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
The first way (`crossterm` crate with feature flags) is preferred. The second way will be
deprecated and no longer supported soon. The `crossterm_*` crates will be marked as deprecated and
repositories archived on the GitHub. See the
[Merge sub-crates to the crossterm crate ](https://github.com/crossterm-rs/crossterm/issues/265 )
for more details.
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
#### `crossterm` crate:
2018-07-31 05:35:35 +10:00
2019-10-02 16:49:01 +10:00
```toml
[dependencies.crossterm]
version = "0.11"
default-features = false # Disable default features
features = ["cursor"] # Enable cursor feature only
2018-07-31 05:35:35 +10:00
```
2019-03-11 10:09:41 +11:00
```rust
2019-10-02 16:49:01 +10:00
use crossterm::cursor;
2019-03-11 10:09:41 +11:00
```
2019-10-02 16:49:01 +10:00
#### `crossterm_curosr` crate:
2019-09-26 00:09:16 +10:00
2019-10-02 16:49:01 +10:00
```toml
[dependencies]
crossterm_cursor = "0.3"
2019-03-11 10:09:41 +11:00
```
```rust
2019-10-02 16:49:01 +10:00
use crossterm_cursor::cursor;
2019-03-11 10:09:41 +11:00
```
2019-10-02 16:49:01 +10:00
### Other Resources
2019-03-11 10:09:41 +11:00
2019-10-02 16:49:01 +10:00
- [API documentation ](https://docs.rs/crossterm/ )
- [Examples repository ](https://github.com/crossterm-rs/examples )
2019-03-11 10:09:41 +11:00
2019-10-02 16:49:01 +10:00
## Used By
2019-09-26 00:09:16 +10:00
2019-06-08 19:28:32 +10:00
- [Broot ](https://dystroy.org/broot/ )
- [Cursive ](https://github.com/gyscos/Cursive )
- [TUI ](https://github.com/fdehau/tui-rs )
- [Rust-sloth ](https://github.com/jonathandturner/rust-sloth/tree/crossterm-port )
2018-07-31 05:35:35 +10:00
## Contributing
2019-10-02 16:49:01 +10:00
I highly appreciate when anyone contributes to this crate. Before you do, please,
read the [Contributing ](docs/CONTRIBUTING.md ) guidelines.
2018-07-31 05:35:35 +10:00
## Authors
* **Timon Post** - *Project Owner & creator*
2019-05-05 05:19:44 +10:00
## Support
2019-10-02 16:49:01 +10:00
Would you like Crossterm to be even more gorgeous and beautiful? You can help with this by donating.
2019-05-05 05:19:44 +10:00
[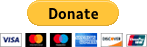](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick& hosted_button_id=Z8QK6XU749JB2)
2018-07-31 05:35:35 +10:00
## License
2019-10-02 16:49:01 +10:00
This project, `crossterm` and all it's sub-crates: `crossterm_screen` , `crossterm_cursor` , `crossterm_style` ,
`crossterm_input` , `crossterm_terminal` , `crossterm_winapi` , `crossterm_utils` are licensed under the MIT
License - see the [LICENSE ](https://github.com/crossterm-rs/crossterm/blob/master/LICENSE ) file for details.
2019-09-26 00:09:16 +10:00
[s1]: https://img.shields.io/crates/v/crossterm.svg
[l1]: https://crates.io/crates/crossterm
[s2]: https://img.shields.io/badge/license-MIT-blue.svg
[l2]: crossterm/LICENSE
[s3]: https://docs.rs/crossterm/badge.svg
[l3]: https://docs.rs/crossterm/
[s3]: https://docs.rs/crossterm/badge.svg
[l3]: https://docs.rs/crossterm/
[s5]: https://img.shields.io/discord/560857607196377088.svg?logo=discord
[l5]: https://discord.gg/K4nyTDB
[s6]: https://tokei.rs/b1/github/crossterm-rs/crossterm?category=code
[s7]: https://travis-ci.org/crossterm-rs/crossterm.svg?branch=master